Rewarded Video Integration for iOS
The ironSource Rewarded Video ad unit offers an engaging ad experience that rewards your users with valuable virtual content in exchange for a completed view. This user-initiated ad unit is great for gaming apps; and enhances every app experience!
Make sure you have correctly integrated the ironSource SDK as well as any additional ad network adapters into your application. Integration is outlined here.
Step 1. Implement the Rewarded Video Delegate
The ironSource SDK fires several events to inform you of ad availability and completions so you’ll know when to reward your users.
The SDK will notify your delegate of all possible events listed below:
+ (void)setLevelPlayRewardedVideoDelegate:(nullable id<LevelPlayRewardedVideoDelegate>)delegate;
#pragma mark - LevelPlayRewardedVideoDelegate
/**
Called after a rewarded video has changed its availability to true.
@param adInfo The info of the ad.
Replaces the delegate rewardedVideoHasChangedAvailability:(true)available
*/
- (void)hasAdAvailableWithAdInfo:(ISAdInfo *)adInfo{
}
/**
Called after a rewarded video has changed its availability to false.
Replaces the delegate rewardedVideoHasChangedAvailability:(false)available
*/
- (void)hasNoAvailableAd{
}
/**
Called after a rewarded video has been viewed completely and the user is eligible for a reward.
@param placementInfo An object that contains the placement's reward name and amount.
@param adInfo The info of the ad.
*/
- (void)didReceiveRewardForPlacement:(ISPlacementInfo *)placementInfo withAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after a rewarded video has attempted to show but failed.
@param error The reason for the error
@param adInfo The info of the ad.
*/
- (void)didFailToShowWithError:(NSError *)error andAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after a rewarded video has been opened.
@param adInfo The info of the ad.
*/
- (void)didOpenWithAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after a rewarded video has been dismissed.
@param adInfo The info of the ad.
*/
- (void)didCloseWithAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after a rewarded video has been clicked.
This callback is not supported by all networks, and we recommend using it
only if it's supported by all networks you included in your build
@param adInfo The info of the ad.
*/
- (void)didClick:(ISPlacementInfo *)placementInfo withAdInfo:(ISAdInfo *)adInfo {
}
You can view the full listeners implementation here.
Error Codes
ironSource provides an error code mechanism to help you understand errors you may run into during integration or live production. See the complete guide here.
Callbacks
Please do not assume the callbacks are always running on the main thread. Any UI interaction or updates resulting from ironSource callbacks need to be passed to the main thread before executing.
Network Connectivity Status
You can determine and monitor the internet connection on the user’s device through the ironSource Network Change Status function. This enables the SDK to change its availability according to network modifications, i.e. in the case of no network connection, the availability will turn to FALSE.The default of this function is false; if you’d like to listen for changes in connectivity, activate it in the SDK initialization with the following string:
[IronSource shouldTrackReachability:YES];[/note]
Step 2. Show a Video Ad to Your Users
Ad Availability
By correctly implementing the Rewarded Video Delegate and its functions, you can receive the availability status through the hasAdAvailableWithAdInfo. You will then be notified with the delegate function below upon ad availability change:
/**
*Called after a rewarded video has changed its availability to true
*@param adInfo The info of the ad.
*/
-(void)hasAdAvailableWithAdInfo:(ISAdInfo *)adInfo{
//Change the in-app 'Traffic Driver' state according to availability.
}
/**
Called after a rewarded video has changed its availability to true
@param adInfo The info of the ad.
*/
public func hasAdAvailable(with adInfo: ISAdInfo) {
//Change the in-app 'Traffic Driver' state according to availability.
}
Alternatively, you can also request ad availability directly by calling:
Ad Placements
With ironSource Ad Placements, you can customize and optimize the Rewarded Video experience. This tool allows you to present videos to users at different locations within your app, and customize the reward amount for each placement. You can use the below function to define the exact place within your app to serve an ad. Navigate to the Ad Placement document for more details.
To get details about the specific reward associated with each ad placement, you can call the following:
ISPlacementInfo *placementInfo = [IronSource rewardedVideoPlacementInfo:(NSString *)placementName];
if(placementInfo != NULL)
{
NSString *rewardName = [placementInfo rewardName];
NSNumber *rewardAmount = [placementInfo rewardAmount];
}
let placementInfo = IronSource.rewardedVideoPlacementInfo(<placementName: String>)
if placementInfo != nil {
let rewardName = placementInfo.placementName
let rewardAmount = placementInfo.rewardAmount
}
Capping & Pacing
In addition to ironSource‘s Ad Placements, you can now configure capping and pacing settings for selected placements. Capping and pacing improves the user experience in your app by limiting the amount of ads served within a defined timeframe. Read more about this feature here.
[IronSource isRewardedVideoCappedForPlacement:@"Placement"];
IronSource.isRewardedVideoCapped(forPlacement: <String>)
When requesting availability, you might receive a TRUE
response but in the case your placement has reached its capping limit, the ad will not be served to the user.
Serve Video Ad
Once an ad network has an available video, you will be ready to show the video to your users. Before you display the ad, make sure to pause any game action, including audio, to ensure the best experience for your users.
[IronSource showRewardedVideoWithViewController:(UIViewController *)viewController placement:(nullable NSString *)placementName];
IronSource.showRewardedVideo(with: <UIViewController>, placement: <String?>)
Dynamic UserID
The Dynamic UserID is a parameter to verify AdRewarded transactions and can be changed throughout the session. To receive this parameter through the server to server callbacks, it must be set before calling showRewardedVideo. You will receive a dynamicUserId parameter in the callback URL with the reward details.
[IronSource setDynamicUserId:@"DynamicUserId"];
IronSource.setDynamicUserId(<dynamicUserId: String>);
Step 3. Reward the User
Each time the user successfully completes a video, the ironSource SDK will fire the didReceiveReward event. Upon reward, you will be notified with the delegate function below.
// Called after a rewarded video has been viewed completely and the user is
// eligible for reward.
// @param placementInfo is an object that contains the placement's reward
// name and amount.
// @param adInfo The info of the ad.
- (void)didReceiveRewardForPlacement:(ISPlacementInfo *)placementInfo withAdInfo:(ISAdInfo *)adInfo {
NSNumber *rewardAmount = [placementInfo rewardAmount];
NSString *rewardName = [placementInfo rewardName];
}
The Placement object contains both the Reward Name & Reward Amount of the Placement as defined in your ironSource Admin:
Server-to-server callbacks
If you turn on server-to-server callbacks, make sure you’ve set the userID before you initialize the ironSource SDK so your users can get rewarded, and make sure to reward your user only once for the same completion.
ironSource LevelPlay will be firing both the client-side callback and the server-to-server callback. So, you will get two notifications in total for each completion.
To utilize server-to-server callbacks, see here.
Done!
You are now all set to deliver Rewarded Video in your application.
If this is a new integration for your application, your app will by default be in ‘Test Mode‘ on your ironSource dashboard. While your app is in Test Mode, the ironSource SDK will print more logs to the console in order to provide greater visibility into the SDK processes. To test your ad inventory, set up your Test Devices. Until you turn on live ad inventory, you will receive test campaigns that don’t generate revenue. Make sure to select Go Live! on the Ad Units page when your app is ready for live ad inventory.
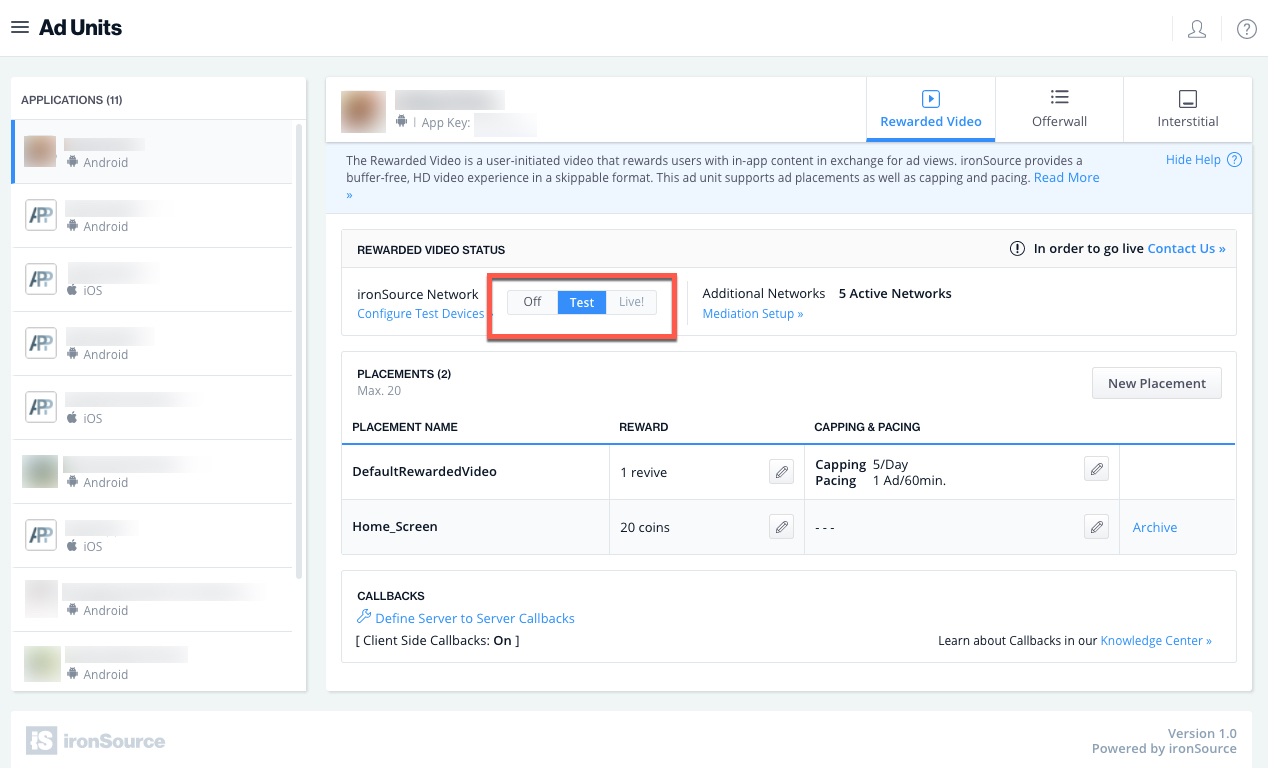
Follow our integration guides to integrate additional Rewarded Video Ad networks or configure additional Ad Units: