iOS에서의 인터스티셜 광고 연동
아이언소스 인터스티셜(전면) 광고는 전체화면 광고 유닛으로, 보통 앱의 생명주기 중 자연스러운 장면 전환 시점에 게재되는 광고입니다. 아이언소스는 정지화면 및 동영상 인터스티셜 광고들 모두를 지원합니다. 또한, 레벨플레이 미디에이션 플랫폼을 통해 인터스티셜 광고를 게재할 수도 있습니다.
1단계. 대리자(Delegate) 구현
아이언소스 SDK는 인터스티셜 동작에 대해 여러 이벤트들을 송출합니다. SDK는 아래에 열거된 가능한 모든 이벤트들에 대한 정보를 대리자(Delegate)를 통해 전달합니다:
+ (void)setLevelPlayInterstitialDelegate:(nullable id<LevelPlayInterstitialDelegate>)delegate;
#pragma mark - LevelPlayInterstitialDelegate
/**
Called after an interstitial has been loaded
@param adInfo The info of the ad.
*/
- (void)didLoadWithAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after an interstitial has attempted to load but failed.
@param error The reason for the error
*/
- (void)didFailToLoadWithError:(NSError *)error {
}
/**
Called after an interstitial has been opened.
This is the indication for impression.
@param adInfo The info of the ad.
*/
- (void)didOpenWithAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after an interstitial has been dismissed.
@param adInfo The info of the ad.
*/
- (void)didCloseWithAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after an interstitial has attempted to show but failed.
@param error The reason for the error
@param adInfo The info of the ad.
*/
- (void)didFailToShowWithError:(NSError *)error andAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after an interstitial has been clicked.
@param adInfo The info of the ad.
*/
- (void)didClickWithAdInfo:(ISAdInfo *)adInfo {
}
/**
Called after an interstitial has been displayed on the screen.
This callback is not supported by all networks, and we recommend using it
only if it's supported by all networks you included in your build.
@param adInfo The info of the ad.
*/
- (void)didShowWithAdInfo:(ISAdInfo *)adInfo {
}
IronSource.setLevelPlayInterstitialDelegate(delegate: LevelPlayInterstitialDelegate?)
// MARK: LevelPlayInterstitialDelegate
/**
Called after an interstitial has been loaded
@param adInfo The info of the ad.
*/
func didLoad(with adInfo: ISAdInfo!) {
}
/**
Called after an interstitial has attempted to load but failed.
@param error The reason for the error
*/
func didFailToLoadWithError(_ error: Error!) {
}
/**
Called after an interstitial has been opened.
This is the indication for impression.
@param adInfo The info of the ad.
*/
func didOpen(with adInfo: ISAdInfo!) {
}
/**
Called after an interstitial has been dismissed.
@param adInfo The info of the ad.
*/
func didClose(with adInfo: ISAdInfo!) {
}
/**
Called after an interstitial has attempted to show but failed.
@param error The reason for the error
@param adInfo The info of the ad.
*/
func didFailToShowWithError(_ error: Error!, andAdInfo adInfo: ISAdInfo!) {
}
/**
Called after an interstitial has been clicked.
@param adInfo The info of the ad.
*/
func didClick(with adInfo: ISAdInfo!) {
}
/**
Called after an interstitial has been displayed on the screen.
This callback is not supported by all networks, and we recommend using it
only if it's supported by all networks you included in your build.
@param adInfo The info of the ad.
*/
func didShow(with adInfo: ISAdInfo!) {
}
전체 대리자(Delegate) 구현은 여기를 참고해 주세요.
2단계. 인터스티셜 광고 로드하기
인터스티셜 광고를 요청하려면 아래의 함수를 호출해 주세요:
3단계. 광고 준비 상태 확인
2단계에서 loadInterstitial을 호출한 다음, didLoad 메서드를 포함한 대리자(Delegate)를 통해 광고가 로딩되고 사용자에게 송출할 준비가 되었음이 이벤트로 전달됩니다.
//Called after an interstitial has been loaded
- (void)didLoadWithAdInfo:(ISAdInfo *)adInfo{}
/**
Called after an interstitial has been loaded
*/
public func didLoad(with adInfo: ISAdInfo) {
}
또는, 아래의 함수를 직접 호출해서 광고 준비 상태를 boolean 값으로 확인할 수도 있습니다. 준비가 되었을 경우 true, 되지 않은 경우 false를 반환합니다:
인터스티셜 광고가 준비되면 사용자에게 광고를 송출할 수 있습니다. 이 때, 레벨플레이 광고 플레이스먼트(게재 위치)을 사용하여 인터스티셜 광고 경험을 커스터마이징 및 최적화 할 수 있습니다. 이 도구를 사용해 인터스티셜 광고를 앱 내의 각기 다른 곳(예: 앱 구동 시, 단계 사이 화면 등)에서 송출할 수 있습니다. 다음 단계의 함수를 사용해서 어느 플레이스먼트에서 광고를 송출할 지 지정할 수 있습니다.
아이언소스의 광고 플레이스먼트기능에서는 특정 플레이스먼트의 광고의 횟수 제한 및 빈도 제한 설정도 할 수 있습니다. 광고의 횟수 및 빈도 제한은 지정된 시간 동안에 게재 가능한 광고의 숫자를 제한하여 사용자의 앱 사용 경험을 향상시킵니다.
사용자에게 인터스티셜 광고를 제공하기 전에 아래와 같이 광고가 게재될 플레이스먼트가 횟수 제한이 있는지 확인해 보시기를 권장 드립니다:
[IronSource isInterstitialCappedForPlacement:placementName];
IronSource.isInterstitialCapped(forPlacement: “placement”)
광고 플레이스먼트(게재 위치) 문서로 이동하시면 상세 정보를 보실 수 있습니다.
4단계. 인터스티셜 광고 송출하기
didLoad콜백을 수신하고 나면, 인터스티셜 광고를 사용자에게 송출할 준비가 완료된 것입니다. 사용자에게 최상의 광고 경험을 제공하기 위해서는 광고가 송출되는 동안 오디오를 비롯한 모든 게임 동작을 일시 정지해 주세요.
다음의 메서드를 호출하여 사용자에게 인터스티셜 광고를 송출하세요:
(void)showInterstitialWithViewController:(UIViewController *)viewController;
IronSource.showInterstitial(with: UIViewController)
만약 인터스티셜 광고에 플레이스먼트(게재 위치)를 설정했다면, 해당 플레이스먼트에 인터스티셜 광고가 송출될 수 있도록 아래의 메서드를 호출하세요:
[IronSource showInterstitialWithViewController:YOUR_VIEWCONTROLLER placement:YOUR_PLACEMENT_NAME]
IronSource.showInterstitial(with: UIViewController, placement: String?)
완료했습니다!
이제 아이언소스 인터스티셜 광고를 레벨플레이 미디에이션 플랫폼을 통해 제공할 수 있습니다!
만약 앱에 처음으로 아이언소스 SDK를 연동하는 경우라면, 이 앱은 아이언소스 대시보드에서 테스트 모드로 기본 설정되어 있을 것입니다. 앱이 테스트 모드일 때에는 아이언소스 SDK는 SDK 처리과정을 좀 더 자세히 볼 수 있도록 더 많은 로그를 콘솔에 송출합니다. 광고 인벤토리 테스트를 위해서는, 테스트 기기를 설정해 주세요. 광고 인벤토리를 라이브 (상용) 모드로 변경하기 전까지는 테스트 캠페인에서 광고를 받고 이 광고들은 수익을 발생시키지 않습니다. 상용 광고 인벤토리의 광고를 앱에 게재할 준비가 되었다면 반드시 레벨플레이 대시보드의 MONETIZE > SETTINGS > Ad Units 페이지에서 Go Live!를 선택해 주세요.

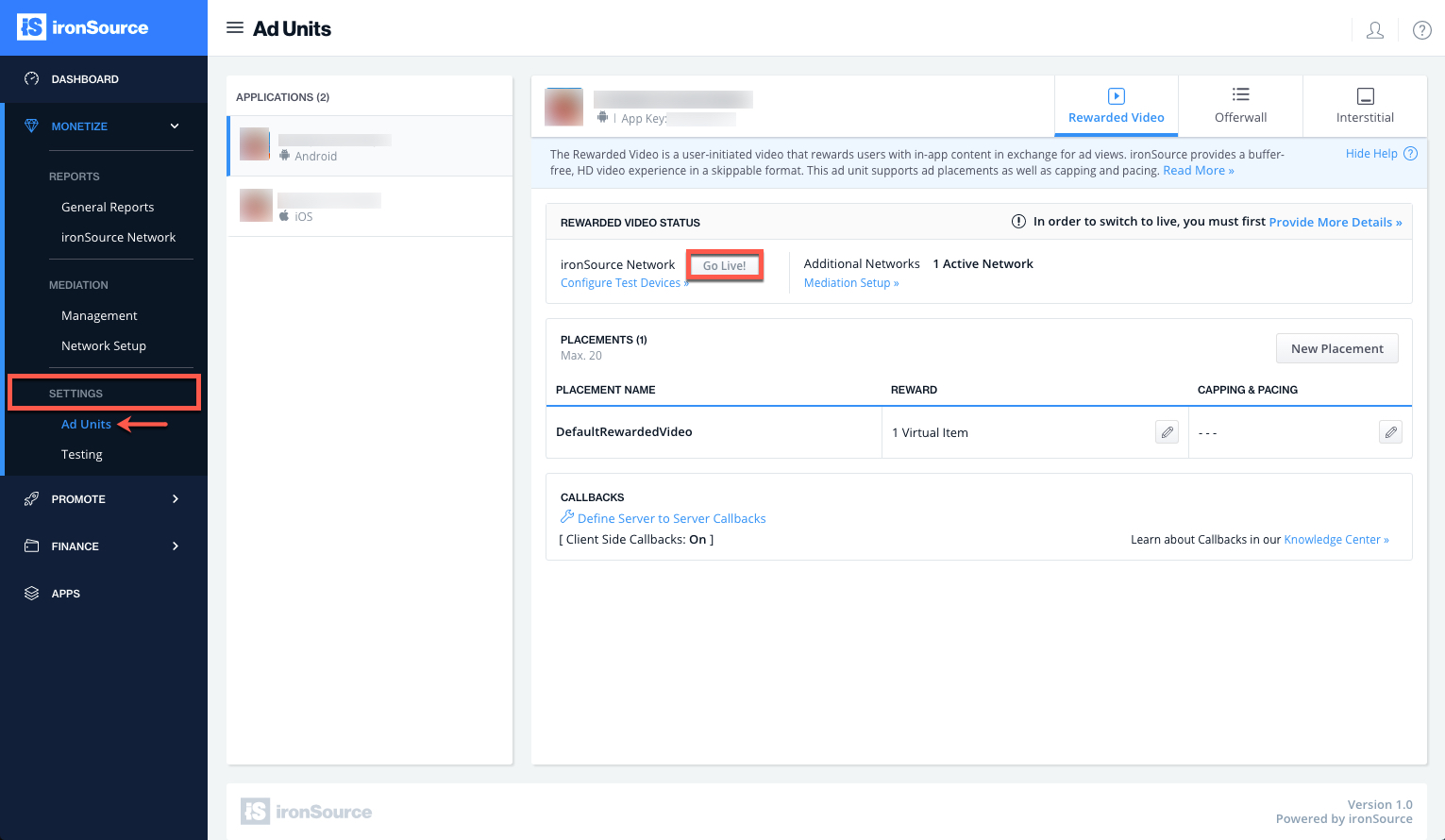
Follow our integration guides to integrate additional Interstitial Ad networks or configure additional ad units: